Introduction
Algorithem is a step by step process to how to solve a program. Algorithm is important because it can influence the speed and efficency of our program. As our program size grow or our users grow, we don’t want slow functions affect our user experience.
Here a sneak peak of some algorithm methods that I’ll go over in further blog series.
- Binary Search
- Fibonacci & Memoized Fibonacci
- Merge Sort & bubble sort
- Caesar Cipher
- Sieve of Eratosthenes
Big O
Lastly I want to talk about Big O notation (or Big O for short). Big O notation is used to classify algorithms according to how their running time or space requirements grow as the input size grows. (source, Wiki). Basically Big O will tell us how scalable a function is?
There are 4 types of Big O and here are some examples
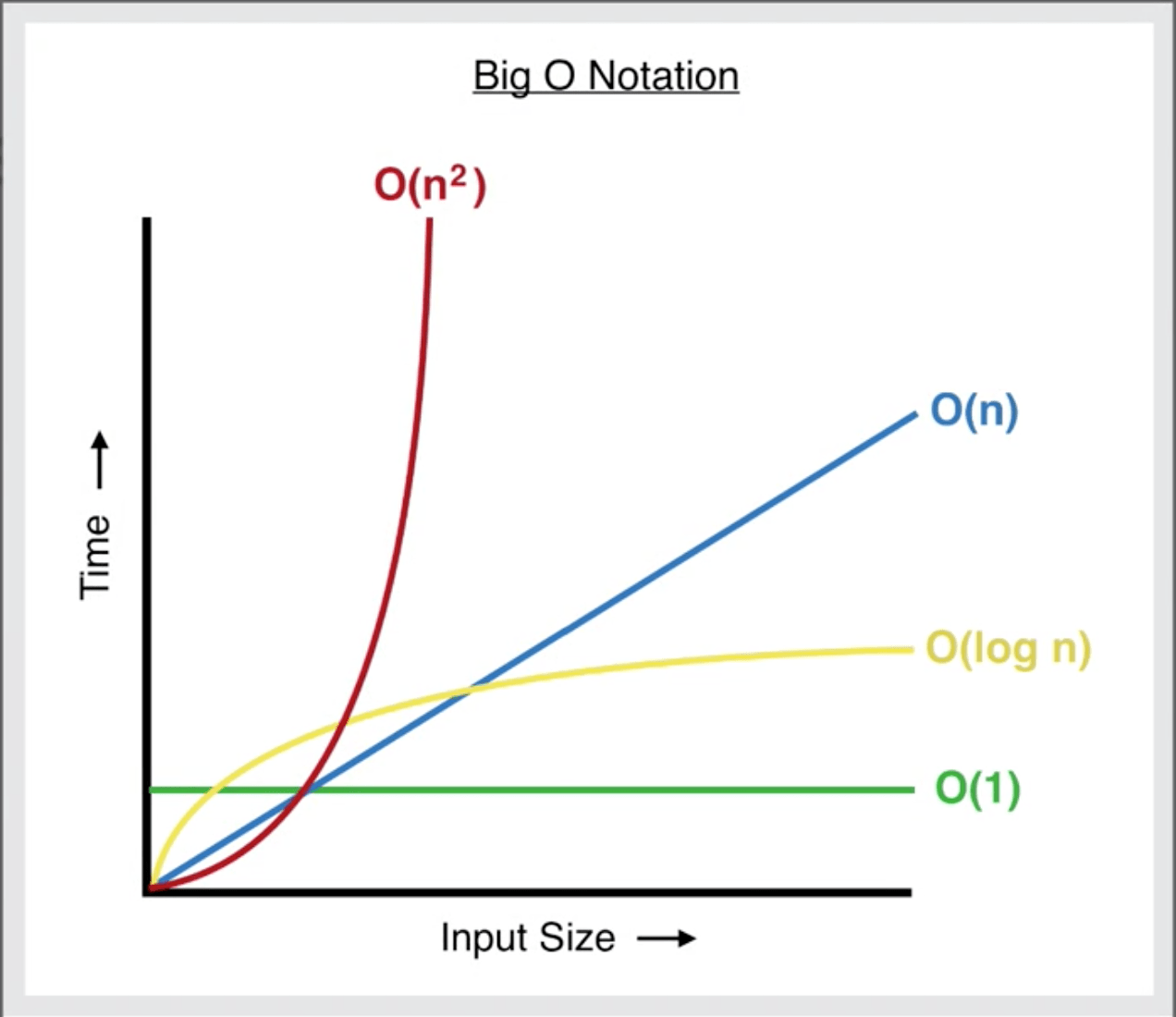
Big O - O (1) - Constant Runtime
|
|
The above example is a constant run time example or sometimes refer as O(1). This means as input size increases on X-axis, the time it takes to run never changes. (Refer to chart Above)
Big O - O (n) - Linear Runtime
|
|
The above example is a linear runtime example or sometimes refer as O(n). This means as input size increases on X-axis, the time will take to run increase linearly.(Refer to chart Above)
Big O - O (n^2) - Exponential Runtime
|
|
The above example is a exponential runtime example or sometimes refer as O(n^2). This means as input size increases on X-axis, the time will take to run increase exponentially.(Refer to chart Above)
We want to stay away from these functions because they are slow and inefficent. They will slow down your application when user grows.
Big O - O (log n) - Logarithmic Runtime
|
|
Binary search takes a list (array) and a key. The array must be sorted in some way (numerically or alphabetically). This method is fast and as the input size grow our execution time will only grow logarithmicly(refer to chart above).
An example of this is looking for a word in dictionary. If we want to look for the word, “House”, we flip to the middle of the dictionary and see how close we are to letter “H”, and we ignore the 2nd half of the dictionary. We keep repeating this process until we are at letter “H”.
A search through 4,000 elements will take 12 operations, and 8000 elements will take 13 operations.